# include file : 외부 소스파일의 내용을 불러오는 기능
ex)
- includeTest.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <meta name="description" content="HTML Study"> <meta name="keywords" content="HTML,CSS,XML,JavaScript"> <meta name="author" content="coderbear"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Insert title here</title> </head> <body> includeTest.jsp 파일입니다. <h1>1. 안녕하세요.</h1> <%@ include file="hello.jsp" %> <h2>2. 반갑습니다.</h2> </body> </html>
- hello.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <% out.print("hello.jsp 입니다."); %>
ex)
- list.jsp
<%@ page import="java.sql.*"%> <%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <meta name="description" content="HTML Study"> <meta name="keywords" content="HTML,CSS,XML,JavaScript"> <meta name="author" content="coderbear"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>게시판 목록</title> </head> <body> <table> <caption>목록</caption> <tr> <td>번호</td><td>제목</td><td>작성자</td><td>작성일</td><td>조회수</td> </tr> <%@ include file="conn/createConnection.jsp" %> <% String sql = "Select no, title, name, wTime, rCnt, email from BOARD ORDER BY no DESC"; try{ pstmt = conn.prepareStatement(sql); rs = pstmt.executeQuery(); while(rs.next()) { %> <tr> <td><%=rs.getInt("no") %></td> <td><a href="view.jsp?no=<%=rs.getInt("no")%>"><%=rs.getString("title") %></a></td> <td><a href="mobileto:email<%=rs.getString("email")%><%=rs.getString("name")%>"></a></td> <td><%=rs.getString("wTime")%></td> <td><%=rs.getString("rCnt")%></td> </tr> <% } } catch(SQLException e){ e.printStackTrace(); } finally{ close(rs, stmt, pstmt, conn); } %> <%@ include file="conn/close.jsp" %> </table> <div><a href="write.jsp">글쓰기</a></div> </body> </html>
- write.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <meta name="description" content="HTML Study"> <meta name="keywords" content="HTML,CSS,XML,JavaScript"> <meta name="author" content="coderbear"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>게시판 글쓰기</title> </head> <body> <form method="post" action="writeOK.jsp"> <table> <caption>글 쓰 기</caption> <tr> <td><label for="title">제목</label></td><td><input type="text" name="title" id="title" size="40" required></td> </tr> <tr> <td><label for="name">이름</label></td><td><input type="text" name="name" id="name" size="40" required></td> </tr> <tr> <td><label for="pw">비밀번호</label></td><td><input type="password" name="pw" id="pw" size="40" required></td> </tr> <tr> <td><label for="email">E-mail</label></td><td><input type="email" name="email" id="email" size="40" required></td> </tr> <tr> <td colspan="2"><textarea cols="50" rows="10" name="contents" required></textarea></td> </tr> <tr> <td colspan="2"><input type="submit" value="등록"> <a href="list.jsp"><input type="button" value="목록"></a></td> </tr> </table> </form> </body> </html>
- writeOK.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <%@ page import="java.sql.*" %> <%@ include file="conn/createConnection.jsp" %> <% request.setCharacterEncoding("UTF-8"); String title = request.getParameter("title"); String name = request.getParameter("name"); String pw = request.getParameter("pw"); String email = request.getParameter("email"); String contents = request.getParameter("contents"); String sql = "INSERT INTO board(title, name, password, email, contents) values(?, ?, ?, ?, ?)"; int result = 0; try{ pstmt = conn.prepareStatement(sql); pstmt.setString(1, title); pstmt.setString(2, name); pstmt.setString(3, pw); pstmt.setString(4, email); pstmt.setString(5, contents); result = pstmt.executeUpdate(); if(result > 0) response.sendRedirect("list.jsp"); else response.sendRedirect("error.jsp"); } catch(SQLException e){ e.printStackTrace(); } finally{ close(rs, stmt, pstmt, conn); } %> <%@ include file="conn/close.jsp" %>
- view.jsp
<%@page import="java.sql.*"%> <%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <meta name="description" content="HTML Study"> <meta name="keywords" content="HTML,CSS,XML,JavaScript"> <meta name="author" content="coderbear"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Insert title here</title> <link rel="stylesheet" href="css/view.css"> </head> <body> <table> <caption>내용보기</caption> <%@ include file="conn/createConnection.jsp" %> <% String no = request.getParameter("no"); String pw = null; String sql = "SELECT title, name, wTime, contents, rCnt, password FROM board where no =" + no; int rCnt = 0; try{ stmt = conn.createStatement(); rs = stmt.executeQuery(sql); while(rs.next()){ rCnt = rs.getInt("rCnt"); pw = rs.getString("password"); %> <tr> <td>제목</td><td><%=rs.getString("title") %></td> </tr> <tr> <td>이름</td><td><%=rs.getString("name") %></td> </tr> <tr> <td>작성일</td><td><%=rs.getString("wTime") %></td> </tr> <tr> <td colspan="2"><textarea name="contents" cols="50" rows="10" style="resize: none;" readonly><%=rs.getString("contents") %></textarea></td> </tr> <% } sql = "UPDATE board SET rCnt = " + (rCnt + 1) + " WHERE no = " + no; stmt.executeUpdate(sql); } catch(SQLException e){ e.printStackTrace(); } finally{ close(rs, stmt, pstmt, conn); } %> <%@ include file="conn/close.jsp" %> </table> <a href="list.jsp"><input type="button" value="목록" class="formbtn"></a> <form method="post" action="inputPassword.jsp"> <input type="hidden" name="no" value="<%=no%>"> <input type="hidden" name="pw" value="<%=pw%>"> <input type="hidden" name="mode" value="2"> <input type="submit" value="수정" class="formbtn"> </form> <form method="post" action="inputPassword.jsp"> <input type="hidden" name="no" value="<%=no%>"> <input type="hidden" name="pw" value="<%=pw%>"> <input type="hidden" name="mode" value="1"> <input type="submit" value="삭제" class="formbtn"> </form> <%-- <a href="inputPassword.jsp?no=<%=no%>&pw=<%=pw%>&mode=1">삭제</a> --%> </body> </html>
- inputPassword.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <meta name="description" content="HTML Study"> <meta name="keywords" content="HTML,CSS,XML,JavaScript"> <meta name="author" content="coderbear"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Insert title here</title> </head> <body> <form method="post" action="inputPasswordOK.jsp"> <table> <tr> <td>비밀번호</td><td><input type="password" name="password"></td> </tr> <tr> <td colspan="2"><input type="submit" value="확인"></td> </tr> </table> <input type = "hidden" name="no" value="<%=request.getParameter("no") %>"> <input type = "hidden" name="pw" value="<%=request.getParameter("pw") %>"> <input type = "hidden" name="mode" value="<%=request.getParameter("mode") %>"> </form> </body> </html>
- inputPasswordOK.jsp
<%@page import="java.sql.*"%> <%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <% String pw = request.getParameter("pw"); String password = request.getParameter("password"); String no = request.getParameter("no"); String mode = request.getParameter("mode"); %> <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <meta name="description" content="HTML Study"> <meta name="keywords" content="HTML,CSS,XML,JavaScript"> <meta name="author" content="coderbear"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Insert title here</title> <script> function goToLocation(no) { if(no==1){ alert("데이터가 삭제 되었습니다"); location.href = "list.jsp"; } else if(no==2){ location.href = "modify.jsp?no=<%=no%>"; } else if(no==3){ alert("비밀번호가 일치하지 않습니다."); history.go(-2); } } </script> </head> <body> <%@ include file="conn/createConnection.jsp" %> <% String sql = null; try{ stmt = conn.createStatement(); if(pw.matches(password)) { if(mode.matches("1")) { sql = "DELETE FROM board where no = " + no; stmt.executeUpdate(sql); %> <script>goToLocation(1)</script> <% } else if(mode.matches("2")) { %> <script>goToLocation(2)</script> <% } } else { %> <script>goToLocation(3)</script> <% } } catch(SQLException e){ e.printStackTrace(); } finally{ close(rs, stmt, pstmt, conn); } %> <%@ include file="conn/close.jsp" %> </body> </html>
- modify.jsp
<%@page import="java.sql.*"%> <%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <meta name="description" content="HTML Study"> <meta name="keywords" content="HTML,CSS,XML,JavaScript"> <meta name="author" content="coderbear"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Insert title here</title> <link rel="stylesheet" href="css/view.css"> </head> <body> <form method="post" action="modifyOK.jsp"> <table> <caption>수정하기</caption> <%@ include file="conn/createConnection.jsp" %> <% String no = request.getParameter("no"); String pw = null; String sql = "SELECT title, name, wTime, contents, rCnt, password FROM board where no =" + no; int rCnt = 0; try{ stmt = conn.createStatement(); rs = stmt.executeQuery(sql); while(rs.next()){ rCnt = rs.getInt("rCnt"); pw = rs.getString("password"); %> <tr> <td>제목</td><td><input type="text" name="title" value="<%=rs.getString("title") %>"></td> </tr> <tr> <td>이름</td><td><%=rs.getString("name") %></td> </tr> <tr> <td>작성일</td><td><%=rs.getString("wTime") %></td> </tr> <tr> <td colspan="2"><textarea name="contents" cols="50" rows="10" style="resize: none;"><%=rs.getString("contents") %></textarea></td> </tr> <% } } catch(SQLException e){ e.printStackTrace(); } finally{ close(rs, stmt, pstmt, conn); } %> <%@ include file="conn/close.jsp" %> </table> <input type="hidden" name="no" value="<%=no %>"> <a href="list.jsp"><input type="button" value="목록"></a> <input type="submit" value="등록"> </form> </body> </html>
- modifyOK.jsp
<%@page import="java.sql.*"%> <%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <%@ include file="conn/createConnection.jsp" %> <% request.setCharacterEncoding("UTF-8"); String no = request.getParameter("no"); String title = request.getParameter("title"); String contents = request.getParameter("contents"); String sql = "UPDATE board SET title=?, contents=? WHERE no = ?"; int result = 0; try{ pstmt = conn.prepareStatement(sql); pstmt.setString(1, title); pstmt.setString(2, contents); pstmt.setInt(3, Integer.parseInt(no)); result = pstmt.executeUpdate(); if(result > 0) response.sendRedirect("list.jsp"); else response.sendRedirect("error.jsp"); } catch(SQLException e){ e.printStackTrace(); } finally{ close(rs, stmt, pstmt, conn); } %> <%@ include file="conn/close.jsp" %>
- error.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <meta name="description" content="HTML Study"> <meta name="keywords" content="HTML,CSS,XML,JavaScript"> <meta name="author" content="coderbear"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Insert title here</title> </head> <body> 에러 </body> </html>
# JavaScript 이벤트 등록
ex)
- Hello.html
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Insert title here</title> </head> <body> Hello.html 입니다.<br> <button onclick="goLocation()">Back</button> <script> function goLocation() { history.go(-1); // 이전 페이지로 이동 } </script> </body> </html>
- JavaScriptTest.html
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Insert title here</title> </head> <body> <button onclick="goLocation()">ClickMe</button> <script> function goLocation() { location.href="Hello.html"; } </script> </body> </html>
- JavaScriptTest2.html
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Insert title here</title> </head> <body> <button id="btn">ClickMe</button> <script> var btn = document.getElementById("btn"); btn.addEventListener('click', goLocation); // 콜백 함수 function goLocation(event) // 이름 없는 함수(anonymous function) { location.href="Hello.html"; } </script> </body> </html>
- JavaScriptTest3.html
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Insert title here</title> </head> <body> <button id="btn">ClickMe</button> <script> var btn = document.getElementById("btn"); btn.addEventListener('click', function(event){ location.href="Hello.html"; }); </script> </body> </html>
# 용어 설명
- API(Application Programming Interface)
OS에서 응용 프로그램을 만들 수 있도록 제공하는 소프트웨어
- 자바 엔터프라이즈 에디션 (Java EE) : 자바를 이용하여 서버 개발을 하기 위한 플랫폼
- WAS(Web Application Server, 웹 어플리케이션 서버)
HTTP를 통해 인터넷 상의 동적 콘텐츠를 수행하는 소프트웨어 엔진
# JSP 내장객체 종류
내장객체 | 사용용도 |
request | 클라이언트 요청에 의한 form 정보처리 |
response | 클라이언트 요청에 대한 응답 |
session | 클라이언트에 대한 세션 정보 처리 |
application | 웹 어플리케이션 정보 처리 |
config | 현재 JSP 페이지에 대한 환경처리 |
exception | 예외처리에 전달되는 객체 |
page | 현재 JSP 페이지에 대한 클래스 정보 |
pageContext | 현재 JSP 페이지에 대한 컨텍스트 |
out | 출력 |
// Tip : exception 내장 객체
page의 isErrorPage 속성이 true일 때만 사용가능함
java.lang.Throwable 객체 타입
+ exception 내장 객체의 메소드
메소드명 | 설명 |
String getMessage() | 발생된 예외의 메시지를 리턴한다 |
String toString() | 발생된 예외 클래스명과 메시지를 리턴한다 |
String printStackTrace() | 발생된 예외를 역추적하기 위해 표준 예외 스트림을 출력한다 예외 발생 시 예외가 발생한 곳을 알아낼 때 주로 사용됨 |
# submit 함수
ex) name 공백 확인 예시
- submitTest.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <meta name="description" content="HTML Study"> <meta name="keywords" content="HTML,CSS,XML,JavaScript"> <meta name="author" content="coderbear"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Insert title here</title> </head> <body> <form name="nameForm" action="SubmitTestOK.jsp" method="post"> 이름 : <input type="text" name="name"><br> <input type="button" onclick="checkName()" value="전송"> // 유효성 체크를 위해 바로 전송하는 것이 아니라 함수를 사용한 것 </form> <script> function checkName(){ var nameForm = document.nameForm; if(nameForm.name.value == ""){ alert("이름을 입력하세요.") }else{ nameForm.submit(); // 서버에 데이터 전송을 위한 함수 } } </script> </body> </html>
- submitTestOK.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <meta name="description" content="HTML Study"> <meta name="keywords" content="HTML,CSS,XML,JavaScript"> <meta name="author" content="coderbear"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Insert title here</title> </head> <body> <% request.setCharacterEncoding("UTF-8"); %> // 한글 사용 가능성을 위해 처리하는 것 이름 : <%= request.getParameter("name") %> </body> </html>
ex) 게시판의 게시물 수 구하기
- CountOfPosts.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <%@ page import="java.sql.*" %> <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <meta name="description" content="HTML Study"> <meta name="keywords" content="HTML,CSS,XML,JavaScript"> <meta name="author" content="coderbear"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Insert title here</title> </head> <body> <% Class.forName("com.mysql.cj.jdbc.Driver"); Connection conn = null; ResultSet rs = null; Statement stmt = null; String sql = "SELECT COUNT(*) FROM BOARD"; int total = 0; try{ conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/project?useSSL=false&serverTimezone=UTC", "root", "1234"); stmt = conn.createStatement(); rs = stmt.executeQuery(sql); if(rs.next()){ total = rs.getInt("COUNT(*)"); System.out.println("게시물 수 " + total); } } catch(SQLException e){ e.printStackTrace(); } %> 게시물 수 <%=total %> </body> </html>
// Tip : JSP 페이지 설정
<%@ page contentType="text/html; charset=XXX" pageEncoding="XXX" %>
① pageEncoding : JSP소스코드의 인코딩(jsp페이지 캐릭터셋)
② contentType 내 charset : HTTP 응답 캐릭터셋 (웹브라우저(HTTP client)가 받아볼 페이지의 캐릭터셋)
HTTP의 헤더에 내용이 들어가게 된다
ex)
<%@ page contentType="text/html; charset=EUC-KR" pageEncoding="UTF-8" %>
JSP파일 : UTF-8로 인코딩
웹브라우저 : EUC-KR로 표현
# Servlet : Java 언어를 기반으로 하는 웹 어플리케이션 기술
+ 실질적으로는 JSP로 화면 구현
- 서블릿 만드는 순서 : NEW - 서블릿 - 크리에이트 서블릿 - NAME, URL mapping - service - finish
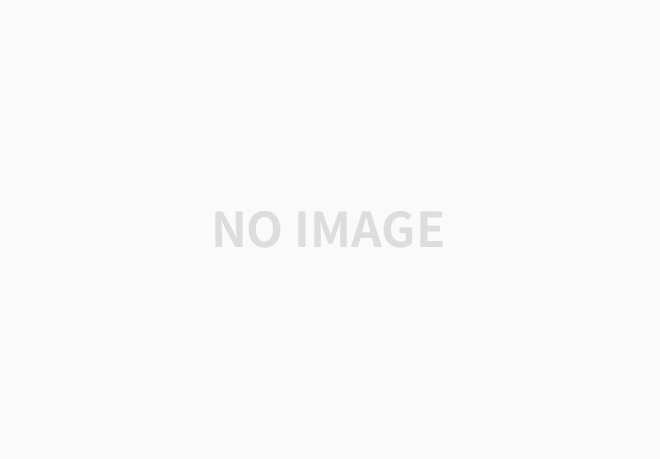
// Tip : 자바 패키지는 회사 도메인명을 반대로 쓰는 경우가 많음
ex)
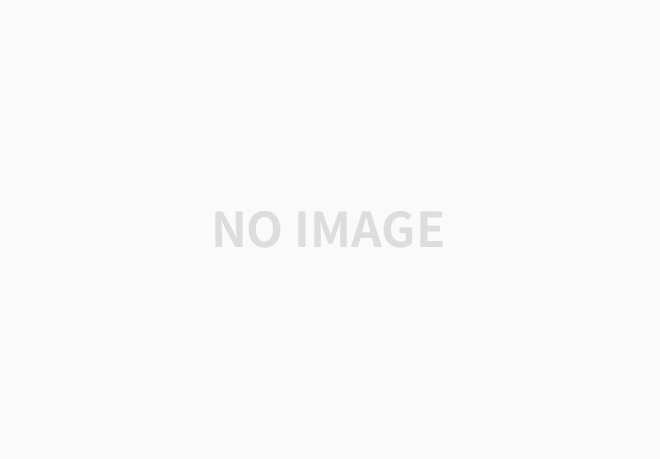
// request, response 매개변수로 받음
// url과 서블릿에서 매핑된 단어가 있는 경우 해당 서블릿으로 가서 내용을 출력함
- setAttribute : 서버에 해당 데이터를 입력해주는 것 (key, value)
value 자리에 컬렉션을 사용할 경우 더 많은 데이터를 입력할 수 있다
- requestDispatcher : 현재 페이지의 request 정보를 저장하여 이동할 페이지로 가더라도 그대로 사용할 수 있는 것
[서블릿/JSP] RequestDispatcher란. RequestDispatcher로 forward() 하기
참고글 [서블릿/JSP] JSP 리다이렉트로 페이지 이동시키기 [서블릿/JSP] JSP 기본객체 종류 [HTTP] 리다이렉트(Redirect)란? RequestDispatcher란 RequestDispatcher는 클라이언트로부터 최초에 들어온 요청을 JSP..
dololak.tistory.com
ex)
- sendRedirect.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <meta name="description" content="HTML Study"> <meta name="keywords" content="HTML,CSS,XML,JavaScript"> <meta name="author" content="Bruce"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Insert title here</title> </head> <body> <% request.setAttribute("name", "홍길동"); response.sendRedirect("result.jsp"); %> </body> </html>
- Result.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <meta name="description" content="HTML Study"> <meta name="keywords" content="HTML,CSS,XML,JavaScript"> <meta name="author" content="Bruce"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Insert title here</title> </head> <body> 이름 : <%=(String)request.getAttribute("name") %> </body> </html>
- RequestDispatcher.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <meta name="description" content="HTML Study"> <meta name="keywords" content="HTML,CSS,XML,JavaScript"> <meta name="author" content="Bruce"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Insert title here</title> </head> <body> <% request.setAttribute("name", "홍길동"); RequestDispatcher dispatcher = request.getRequestDispatcher("result.jsp"); dispatcher.forward(request, response); %> </body> </html>
Q. 두 개의 이미지와 같이 Servlet을 각각 구현하시오.
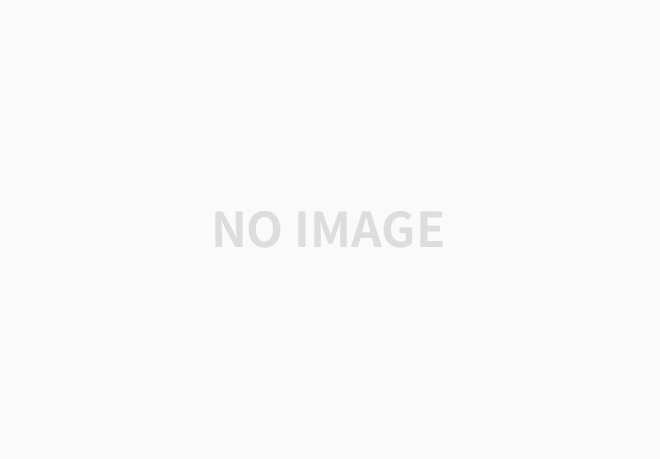
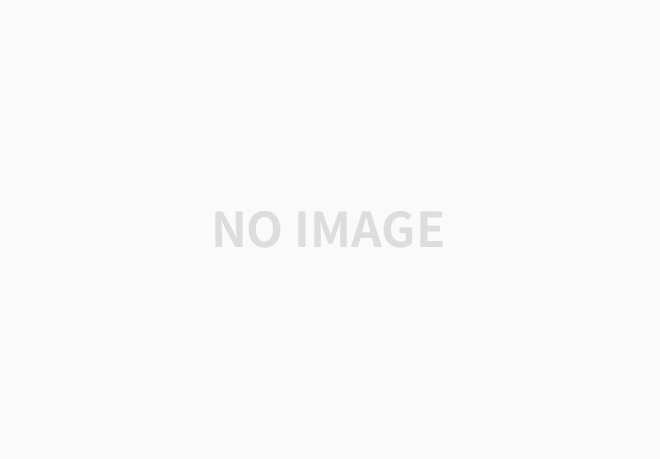
A.
- ServletTest.java
package com.tistory.coderbear; import java.io.*; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; @WebServlet("/ServletTest") public class ServletTest extends HttpServlet { private static final long serialVersionUID = 1L; public ServletTest() { super(); } protected void service(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { response.setContentType("text/html;charset=utf-8"); PrintWriter out = response.getWriter(); out.println("<!DOCTYPE html>"); out.println("<html>"); out.println("<head>"); out.println("<title>servletTest</title>"); out.println("</head>"); out.println("<body>"); out.println("<form action='ServletResult'>"); out.println("<table border='1'>"); out.println("<tr>"); out.println("<td>이름</td>"); out.println("<td><input type='text' name='name'></td>"); out.println("</tr>"); out.println("<tr>"); out.println("<td>아이디</td>"); out.println("<td><input type='text' name='id'></td>"); out.println("</tr>"); out.println("<tr>"); out.println("<td>비밀번호</td>"); out.println("<td><input type='password' name='password'></td>"); out.println("</tr>"); out.println("<tr>"); out.println("<td>취미</td>"); out.println("<td>"); out.println("<input type='checkbox' name='hobby' value='reading' id='reading'><label for='reading'>독서</label>"); out.println("<input type='checkbox' name='hobby' value='cooking' id='cooking'><label for='cooking'>요리</label>"); out.println("<input type='checkbox' name='hobby' value='jogging' id='jogging'><label for='jogging'>조깅</label>"); out.println("<input type='checkbox' name='hobby' value='swimming' id='swimming'><label for='swimming'>수영</label>"); out.println("<input type='checkbox' name='hobby' value='sleeping' id='sleeping'><label for='sleeping'>취침</label>"); out.println("</td>"); out.println("</tr>"); out.println("<tr>"); out.println("<td>"); out.println("전공"); out.println("</td>"); out.println("<td>"); out.println("<input type='radio' name='major' value='kor' id='kor'><label for='kor'>국어</label>"); out.println("<input type='radio' name='major' value='eng' id='eng'><label for='eng'>영어</label>"); out.println("<input type='radio' name='major' value='math' id='math'><label for='math'>수학</label>"); out.println("<input type='radio' name='major' value='design' id='design'><label for='design'>디자인</label>"); out.println("</td"); out.println("</tr>"); out.println("<tr>"); out.println("<td>"); out.println("프로토콜"); out.println("</td>"); out.println("<td>"); out.println("<select name='protocol'>"); out.println("<option value='http'>http</option>"); out.println("<option value='ftp' selected>ftp</option>"); out.println("<option value='smtp'>smtp</option>"); out.println("<option value='pop'>pop</option>"); out.println("</select>"); out.println("</td>"); out.println("</tr>"); out.println("<tr>"); out.println("<td colspan='2'>"); out.println("<input type='submit' value='전송'> "); out.println("<input type='reset' value='초기화'> "); out.println("</td>"); out.println("</tr>"); out.println("</table>"); out.println("</form>"); out.println("</body>"); out.println("</html>"); } }
- ServletResult.java
package com.tistory.coderbear; import java.io.IOException; import java.io.PrintWriter; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; @WebServlet("/ServletResult") public class ServletResult extends HttpServlet { private static final long serialVersionUID = 1L; public ServletResult() { super(); } protected void service(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { response.setContentType("text/html;charset=utf-8"); PrintWriter out = response.getWriter(); out.println("<!DOCTYPE html>"); out.println("<html>"); out.println("<head>"); out.println("<title>ServeletResult</title>"); out.println("</head>"); out.println("<body>"); out.println("이름 : " + request.getParameter("name") +"<br>"); out.println("아이디 : " + request.getParameter("id") +"<br>"); out.println("비밀번호 : " + request.getParameter("password") +"<br>"); out.println("취미 : ["); String[] hobby = request.getParameterValues("hobby"); for(int i=0;i<hobby.length;i++) { out.println(hobby[i]); if(i!=hobby.length-1)out.println(", "); } out.println("]<br>"); out.println("전공 : " + request.getParameter("major") +"<br>"); out.println("프로토콜 : " + request.getParameter("protocol") +"<br>"); out.println("</body>"); out.println("</html>"); } }